perlunless
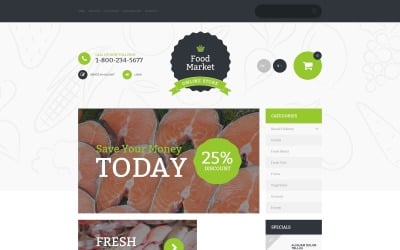
Perl is a high-level
general-purpose programming language that was created by Larry Wall in the late 1980s. It is known for its flexibility
powerful text processing capabilities
and extensive library of modules that make it ideal for a wide range of applications. One of the key features of Perl is its ability to handle complex data structures
which makes it a popular choice for tasks such as data manipulation
text processing
and web development.
One of the unique features of Perl is the "unless" statement
which is used to execute a block of code only if a certain condition is false. The syntax of the "unless" statement is similar to that of the "if" statement
but with the condition reversed. For example:
```
unless ($x > 10) {
print "x is not greater than 10";
}
```
In this example
the block of code will only be executed if the value of $x is not greater than 10. If $x is greater than 10
the code within the block will not be executed.
The "unless" statement can also be used with an "else" block
which will be executed if the condition is true. For example:
```
unless ($x > 10) {
print "x is not greater than 10";
} else {
print "x is greater than 10";
}
```
In this example
if $x is not greater than 10
the first block of code will be executed. If $x is greater than 10
the second block of code will be executed.
The "unless" statement can also be used with "elsif" blocks to check multiple conditions. For example:
```
unless ($x > 10) {
print "x is not greater than 10";
} elsif ($x == 10) {
print "x is equal to 10";
} else {
print "x is greater than 10";
}
```
In this example
the first block of code will be executed if $x is not greater than 10. If $x is equal to 10
the second block of code will be executed. If $x is greater than 10
the third block of code will be executed.
Overall
the "unless" statement in Perl provides a convenient way to execute code based on the negation of a condition. It is a useful tool for writing clear and concise code
especially in cases where the condition is more naturally expressed in a negative form.