vue数组操作
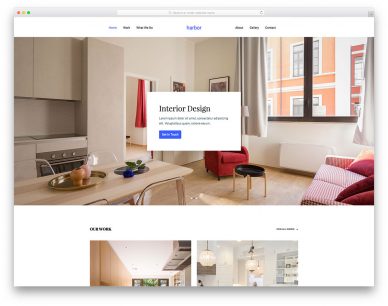
Vue中的数组操作非常灵活,可以进行增加、删除、修改、查找等操作。在Vue中,我们可以通过使用内置的数组方法或通过自定义方法来实现对数组的操作。
一、数组的创建和初始化
在Vue中,我们可以使用响应式数据进行数组的创建和初始化。例如:
```javascript
data() {
return {
fruits: ['apple'
'banana'
'orange']
}
}
```
二、获取数组的长度
可以使用length属性获取数组的长度。例如:
```javascript
console.log(this.fruits.length); // 3
```
三、访问数组元素
可以使用索引来访问数组中的元素,索引从0开始计数。例如:
```javascript
console.log(this.fruits[0]); // apple
```
四、添加元素到数组末尾
可以使用push方法将元素添加到数组的末尾。例如:
```javascript
this.fruits.push('grape');
console.log(this.fruits); // ['apple'
'banana'
'orange'
'grape']
```
五、删除数组末尾的元素
可以使用pop方法删除数组末尾的元素。例如:
```javascript
this.fruits.pop();
console.log(this.fruits); // ['apple'
'banana'
'orange']
```
六、添加元素到数组开头
可以使用unshift方法将元素添加到数组的开头。例如:
```javascript
this.fruits.unshift('grape');
console.log(this.fruits); // ['grape'
'apple'
'banana'
'orange']
```
七、删除数组开头的元素
可以使用shift方法删除数组开头的元素。例如:
```javascript
this.fruits.shift();
console.log(this.fruits); // ['apple'
'banana'
'orange']
```
八、删除指定位置的元素
可以使用splice方法删除指定位置的元素。例如:
```javascript
this.fruits.splice(1
1);
console.log(this.fruits); // ['apple'
'orange']
```
九、修改指定位置的元素
可以通过索引来修改指定位置的元素。例如:
```javascript
this.fruits[1] = 'grape';
console.log(this.fruits); // ['apple'
'grape'
'orange']
```
十、查找元素在数组中的位置
可以使用indexOf方法查找元素在数组中的位置。例如:
```javascript
console.log(this.fruits.indexOf('apple')); // 0
```
总结:
通过以上的介绍,我们可以看到Vue中的数组操作非常简单,可以实现数组的增删改查等常见操作。在实际开发中,我们可以根据具体的需求选择合适的数组方法来实现对数组的操作。同时,我们也可以通过自定义方法来进一步扩展数组操作的功能。Vue的数组操作灵活性高,简化了开发过程,提高了开发效率。