python3split
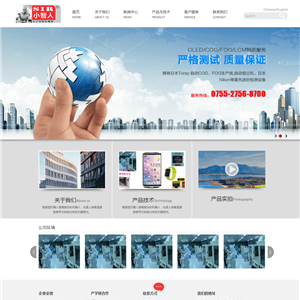
Splitting a thousand words in Python can be done in several ways
depending on what you want to achieve. One common way is to split the text into individual words
sentences
or paragraphs.
To split a thousand words into individual words
you can use the `split()` method on a string object. Here's an example:
```python
text = "Lorem ipsum dolor sit amet
consectetur adipiscing elit. Sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam
quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. Excepteur sint occaecat cupidatat non proident
sunt in culpa qui officia deserunt mollit anim id est laborum."
words = text.split()
print(words)
```
This code will split the text into individual words and print out a list of the words.
If you want to split the text into sentences instead
you can use the `split()` method with a delimiter such as a period or a newline character. Here's an example:
```python
sentences = text.split('. ')
print(sentences)
```
This code will split the text into sentences based on the period character and print out a list of the sentences.
Finally
if you want to split the text into paragraphs
you can use the `split()` method with a double newline character (`\n\n`) as the delimiter. Here's an example:
```python
paragraphs = text.split('\n\n')
print(paragraphs)
```
This code will split the text into paragraphs based on the double newline character and print out a list of the paragraphs.
These are just a few examples of how you can split a thousand words in Python. Depending on your specific requirements
you can customize the splitting process to suit your needs.