oslistdir
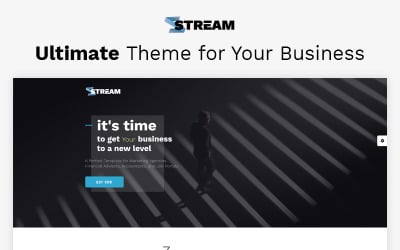
os.listdir() is a function in Python that is used to obtain a list of files and subdirectories in a specific directory. This function is a part of the os module in Python
which provides a way to interact with the operating system.
To use os.listdir()
you first need to import the os module in your Python script. Then
you can call the os.listdir() function with the path of the directory as its argument. The function will return a list of all the files and subdirectories present in the specified directory.
For example
if you have a directory named "test_directory" with the following files and subdirectories:
- file1.txt
- file2.txt
- subdirectory1
- subdirectory2
You can use os.listdir() to obtain a list of these files and subdirectories like this:
```python
import os
directory = "test_directory"
items = os.listdir(directory)
for item in items:
print(item)
```
When you run this script
you will get the following output:
file1.txt
file2.txt
subdirectory1
subdirectory2
It is important to note that os.listdir() only returns the names of the files and subdirectories in the specified directory
not their full paths. If you need the full paths
you can use os.path.join() to join the directory path with the item name.
Additionally
os.listdir() does not list hidden files or directories by default. To include hidden files and directories in the list
you can use os.listdir('.') instead of os.listdir(directory).
In conclusion
os.listdir() is a useful function in Python for obtaining a list of files and subdirectories in a specified directory. It is commonly used in file management tasks and directory traversal operations.