array_splice
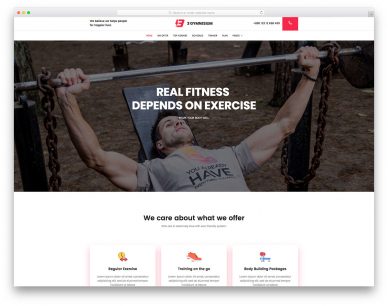
array_splice is a built-in PHP function that is used to remove a portion of an array and replace it with new elements if needed. It is a versatile function that can perform a wide range of operations on arrays
making it a valuable tool for developers working with PHP.
One common use of array_splice is to remove a specific range of elements from an array. This can be done by specifying the start index and the length of the elements to be removed. For example
if we have an array called $colors containing various color names
we can use array_splice to remove a specific range of colors from the array.
```php
$colors = array("red"
"blue"
"green"
"yellow"
"purple");
// Remove elements starting from index 1 and remove 2 elements
array_splice($colors
1
2);
// Output: Array ( [0] => red [2] => purple )
print_r($colors);
```
In this example
array_splice removed the elements "blue" and "green" from the array $colors starting from index 1. The resulting array only contains "red" and "purple".
Another common use of array_splice is to insert new elements into an array at a specific position. This can be done by specifying the start index
the number of elements to remove (which can be 0)
and the new elements to be inserted. For example
if we want to insert the color "orange" into the $colors array at index 2
we can use array_splice to achieve this.
```php
$colors = array("red"
"blue"
"green"
"yellow"
"purple");
// Insert "orange" at index 2
array_splice($colors
2
0
"orange");
// Output: Array ( [0] => red [1] => blue [2] => orange [3] => green [4] => yellow [5] => purple )
print_r($colors);
```
In this example
array_splice inserted the color "orange" into the $colors array at index 2 without removing any existing elements. The resulting array now contains "orange" at the specified position.
Furthermore
array_splice can also be used to replace elements in an array with new elements. This can be done by specifying the start index
the number of elements to remove
and the new elements to be inserted in place of the removed elements. For example
if we want to replace the colors "green" and "yellow" in the $colors array with "pink" and "brown"
we can use array_splice to achieve this.
```php
$colors = array("red"
"blue"
"green"
"yellow"
"purple");
// Replace "green" and "yellow" with "pink" and "brown"
array_splice($colors
2
2
array("pink"
"brown"));
// Output: Array ( [0] => red [1] => blue [2] => pink [3] => brown [4] => purple )
print_r($colors);
```
In this example
array_splice replaced the colors "green" and "yellow" in the $colors array with "pink" and "brown" respectively. The resulting array now contains the new elements at the specified positions.
In conclusion
array_splice is a powerful function in PHP that allows developers to manipulate arrays by removing
inserting
or replacing elements easily. It is a versatile tool that can be used in various scenarios to perform complex operations on arrays efficiently. Whether you need to remove specific elements
insert new elements
or replace existing elements
array_splice can help you achieve your desired array manipulation tasks with ease.