pythonsuper()
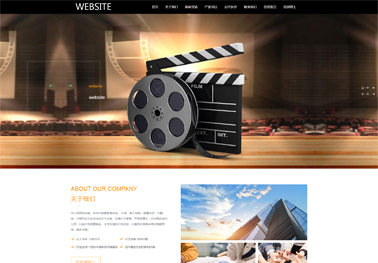
PythonSuper() is a built-in function in Python that allows us to access attributes and methods from a superclass in a subclass. This function is commonly used in object-oriented programming to achieve inheritance and code reusability.
When we create a subclass in Python
we can use the super() function to call the superclass's constructor and methods. This helps in avoiding code duplication and promotes a clean and organized code structure.
The super() function takes two parameters: the subclass itself and the instance of the subclass. By using super()
we can access the superclass's methods and attributes without explicitly referring to the superclass's name.
Here is an example to demonstrate the usage of the super() function in Python:
```python
class Animal:
def __init__(self
name):
self.name = name
def speak(self):
print("Animal speaks")
class Dog(Animal):
def __init__(self
name
breed):
super().__init__(name)
self.breed = breed
def speak(self):
super().speak()
print("Dog barks")
# Creating an instance of the Dog class
dog = Dog("Buddy"
"Golden Retriever")
# Calling the speak method of the Dog class
dog.speak()
```
In the example above
the Dog class inherits from the Animal class. In the Dog class's constructor
we use super() to call the superclass's constructor with the name parameter. In the speak method of the Dog class
we use super() to call the superclass's speak method before printing "Dog barks".
Using super() in this way allows us to maintain a clear hierarchy of classes and promotes the reuse of code. It also makes the code more maintainable and easier to understand.
In conclusion
the super() function in Python is a powerful tool that enables us to work with inheritance and superclass methods seamlessly. By using super()
we can create efficient and organized code structures that are easy to maintain and extend.