.pop()
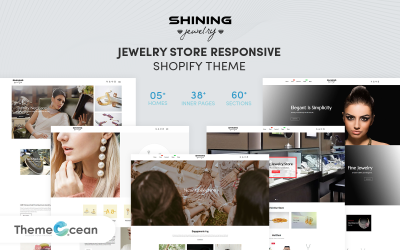
.pop() is a built-in method in Python that is used to remove and return the last item from a list. It can be a useful tool for managing and manipulating lists in Python. In this article
we will explore the .pop() method in detail and see how it can be used in different scenarios.
The .pop() method takes an optional argument
which is the index of the item to be removed from the list. If no index is specified
the method will remove and return the last item in the list. Let's see some examples to understand how the .pop() method works:
```python
# Example 1: Remove and return the last item from a list
fruits = ['apple'
'banana'
'cherry'
'orange']
last_fruit = fruits.pop()
print(last_fruit) # Output: orange
print(fruits) # Output: ['apple'
'banana'
'cherry']
# Example 2: Remove and return the item at a specific index
colors = ['red'
'green'
'blue'
'yellow']
color = colors.pop(2)
print(color) # Output: blue
print(colors) # Output: ['red'
'green'
'yellow']
```
As you can see from the examples above
the .pop() method can be used to remove and return items from a list with ease. It is especially useful when you need to access and work with the last item in a list
or when you want to remove an item at a specific index.
The .pop() method modifies the original list by removing the item that is popped. So if you need to keep the original list intact
you should make a copy of the list before using the .pop() method. For example:
```python
original_list = ['a'
'b'
'c'
'd']
new_list = list(original_list)
item = new_list.pop()
print(item) # Output: d
print(original_list) # Output: ['a'
'b'
'c']
print(new_list) # Output: ['a'
'b'
'c']
```
In this example
we create a copy of the original list before removing an item using the .pop() method. This ensures that the original list remains unchanged
while the new list contains the popped item.
It is important to note that the .pop() method raises an IndexError if you try to pop an item from an empty list or if the index specified is out of range. To avoid this error
you can use a conditional statement to check if the list is empty before using the .pop() method. For example:
```python
my_list = []
if my_list:
item = my_list.pop()
print(item)
else:
print("List is empty")
```
In this example
we first check if the list is empty before using the .pop() method. If the list is empty
we print a message indicating that the list is empty.
In conclusion
the .pop() method is a useful tool for removing and returning items from a list in Python. It can be used to access the last item in a list or remove an item at a specific index. Just remember to handle cases where the list is empty or the index is out of range to avoid errors.