pythonint()
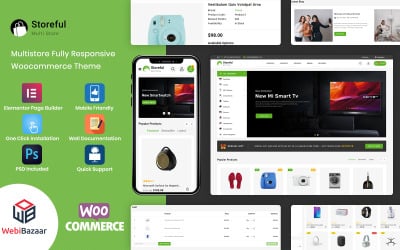
int() function in Python is used to convert a number or a string that represents a number into an integer. It removes any decimal part and returns the integer value.
To use the int() function in Python
you can simply write int() followed by the number or string that you want to convert into an integer. For example:
```
num_str = "10"
num_int = int(num_str)
print(num_int)
```
This will output:
```
10
```
If you try to convert a string that cannot be converted into an integer using the int() function
you will get a ValueError. For example:
```
num_str = "abc"
num_int = int(num_str)
```
This will raise a ValueError.
You can also convert floating-point numbers to integers using the int() function. The function will truncate the decimal part of the number. For example:
```
float_num = 10.5
int_num = int(float_num)
print(int_num)
```
This will output:
```
10
```
If you want to convert a binary
octal
or hexadecimal number to an integer
you can use the int() function with the base argument. The base argument specifies the base of the number. For example
to convert a binary number to an integer:
```
binary_num = "1010"
int_num = int(binary_num
2)
print(int_num)
```
This will output:
```
10
```
Similarly
you can convert an octal or hexadecimal number to an integer by specifying the base as 8 or 16
respectively.
In conclusion
the int() function in Python is a versatile function that allows you to convert numbers or strings representing numbers into integers. It is a useful tool for data manipulation and mathematical operations in Python programming.