htmlsession
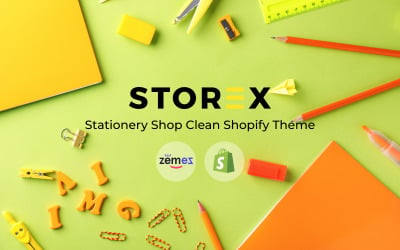
HTMLSession is a utility class provided by the `httpx` library in Python. It is used for making HTTP requests and managing sessions in an efficient manner. In this article
we will explore the functionalities and capabilities of HTMLSession in detail.
HTTP (Hypertext Transfer Protocol) is the underlying protocol used for communication on the World Wide Web. When we interact with a website
our web browser makes multiple HTTP requests to fetch various resources like HTML pages
images
scripts
etc. HTMLSession provides a convenient way to make these HTTP requests in Python.
To get started with HTMLSession
we first need to install the `httpx` library. It can be installed using the pip package manager by running the following command:
```
pip install httpx
```
Once installed
we can import the necessary classes and functions by adding the following line at the beginning of our Python script:
```python
from httpx import HTMLSession
```
Now
let's explore the various features and functionalities of HTMLSession:
1. Making HTTP Requests:
HTMLSession provides various methods for making HTTP requests - `get()`
`post()`
`put()`
`delete()`
etc. These methods accept a URL as input and return a `Response` object containing the server's response. For example
to make a GET request:
```python
session = HTMLSession()
response = session.get('https://www.example.com')
```
The `response` object contains various attributes like `status_code` (HTTP status code)
`text` (response content)
`json()` (parsed JSON response)
etc.
HTMLSession also supports asynchronous requests using the `async_get()`
`async_post()`
etc. methods. We can use the `await` keyword to asynchronously wait for the response.
2. Session Management:
HTMLSession automatically manages cookies and other session-related information. Once we create an HTMLSession object
it uses the same session for subsequent requests
allowing us to maintain state across multiple requests. For example
if we authenticate with a website
the session will automatically store the necessary cookies for future requests.
```python
session = HTMLSession()
response = session.post('https://www.example.com/login'
data={'username': 'user'
'password': 'pass'})
```
We can then use the same `session` object for subsequent requests
and it will include the necessary cookies for authentication.
3. Handling Redirects:
When we make an HTTP request
the server may respond with a redirect status code (e.g.
302 Found). HTMLSession automatically handles these redirects and returns the final response. We don't need to manually follow redirects or modify the request.
4. Custom Headers and Authentication:
HTMLSession allows us to send custom headers with our requests. This can be useful for providing authentication tokens
user-agent strings
or other custom data. We can pass a `headers` parameter to the request methods.
```python
session = HTMLSession()
headers = {'User-Agent': 'Mozilla/5.0'}
response = session.get('https://www.example.com'
headers=headers)
```
5. Session Timeout and Retry:
HTMLSession supports setting a timeout for requests. If a response is not received within the specified timeout period
it raises a `TimeoutException`. We can also configure retry logic for failed requests using the `max_retries` parameter.
```python
session = HTMLSession(timeout=10
max_retries=3)
response = session.get('https://www.example.com')
```
6. Proxy Support:
HTMLSession can make requests through a proxy server by specifying the `proxies` parameter. This is useful for scenarios where we want to hide our IP address or bypass network restrictions.
```python
proxies = {'http': 'http://your-proxy-server:port'
'https': 'http://your-proxy-server:port'}
session = HTMLSession(proxies=proxies)
response = session.get('https://www.example.com')
```
In conclusion
HTMLSession is a powerful and flexible utility class for making HTTP requests and managing sessions in Python. It simplifies the process of interacting with websites and provides a variety of features for customizing requests and handling responses. By using HTMLSession
we can easily build web scraping tools
interact with APIs
or automate web-based tasks in our Python projects.