pythonmath.log
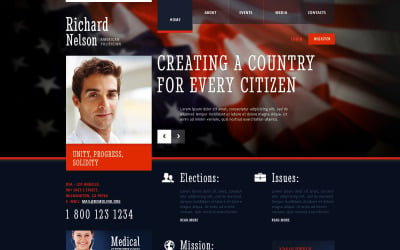
`math.log()` is a Python function that calculates the natural logarithm of a given number. The natural logarithm is the logarithm to the base 'e'
where 'e' is the mathematical constant approximately equal to 2.71828.
To use the `math.log()` function in Python
you need to import the `math` module at the beginning of your script. This can be done by including the following line of code:
```python
import math
```
Once you have imported the `math` module
you can use the `math.log()` function to calculate the natural logarithm of a number. The function takes two arguments: the number you want to find the logarithm of
and the base of the logarithm (which is 'e' for the natural logarithm).
Here is an example of how to use the `math.log()` function in Python:
```python
import math
number = 10
result = math.log(number)
print(f"The natural logarithm of {number} is {result}")
```
When you run this code
the output will be:
```
The natural logarithm of 10 is 2.302585092994046
```
The `math.log()` function is useful for various mathematical calculations
such as calculating exponential growth or decay
determining the time it takes for a quantity to double or halve
and solving equations involving logarithms.
In summary
the `math.log()` function in Python is a powerful tool for calculating natural logarithms
and it can be used in a variety of mathematical contexts.