vue文件上传
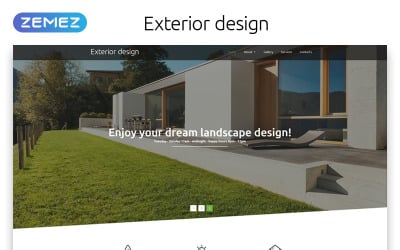
Vue文件上传是指使用Vue框架来实现文件上传功能。文件上传是Web开发中常见的需求,它允许用户选择文件并将其上传到服务器。在Vue中,可以利用Vue的组件化开发思想和axios库来轻松实现文件上传。
Vue文件上传的基本思路是,首先在Vue组件中添加一个文件选择框,让用户可以选择要上传的文件。然后通过事件监听等方式获取用户选择的文件,并使用axios库将文件上传到服务器。在上传过程中,可以显示进度条,上传成功后可显示上传结果。
为了实现文件上传功能,可以在Vue组件中添加以下代码:
```html
上传中...
import axios from 'axios';
export default {
data() {
return {
file: null
uploading: false
uploadProgress: 0
}
}
methods: {
handleFileChange(event) {
this.file = event.target.files[0];
}
uploadFile() {
const formData = new FormData();
formData.append('file'
this.file);
this.uploading = true;
this.uploadProgress = 0;
axios.post('/api/upload'
formData
{
headers: {
'Content-Type': 'multipart/form-data'
}
onUploadProgress: (progressEvent) => {
this.uploadProgress = Math.round((progressEvent.loaded / progressEvent.total) * 100);
}
}).then(() => {
this.uploading = false;
this.file = null;
this.uploadProgress = 0;
alert('上传成功!');
}).catch(() => {
this.uploading = false;
alert('上传失败!');
});
}
}
}
```
在上述代码中,``元素用于选择要上传的文件,`@change`事件监听文件选择框的变化,当用户选择文件后,`handleFileChange`方法会将选择的文件存储在`file`属性中。
`