vue防抖
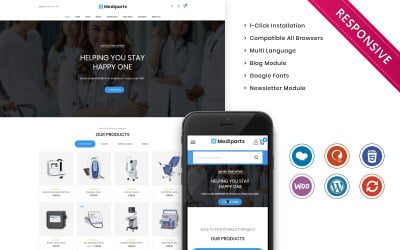
Vue框架中的防抖技术是指在某个事件被触发后,延迟一段时间再执行该事件的回调函数。这个延迟时间可以自定义,一般用于解决频繁触发的事件导致性能问题的情况。
在Vue中,我们可以通过自定义指令、mixin或者在组件内部直接使用debounce来实现防抖效果。下面将详细介绍这几种实现方式。
1. 使用自定义指令实现防抖
自定义指令是Vue中常用的扩展方式之一,通过在指令的bind函数中添加防抖逻辑,在事件被触发时延迟执行回调函数。
```javascript
// 防抖指令
Vue.directive('debounce'
{
bind: function (el
binding) {
let timer = null;
el.addEventListener('click'
function () {
if (timer) {
clearTimeout(timer);
}
timer = setTimeout(() => {
binding.value();
}
binding.arg || 200);
});
}
})
```
然后在组件中使用v-debounce指令即可:
```html
export default {
methods: {
handleClick() {
console.log('按钮点击事件');
}
}
}
```
2. 使用mixin实现防抖
mixin是一种在多个组件中复用方法的方式,可以将防抖的逻辑封装成一个mixin,并全局注入所有的组件。
```javascript
// 防抖mixin
const debounceMixin = {
methods: {
debounce(func
delay = 200) {
let timer = null;
return function () {
if (timer) {
clearTimeout(timer);
}
timer = setTimeout(() => {
func.apply(this
arguments);
}
delay);
}
}
}
}
// main.js 全局注入mixin
import debounceMixin from './debounceMixin';
Vue.mixin(debounceMixin);
```
然后在组件内部使用this.debounce方法即可:
```html
export default {
methods: {
handleClick() {
console.log('按钮点击事件');
}
}
}
```
3. 在组件内部直接使用debounce
如果只需要在某个组件内部使用防抖功能,我们可以直接在组件中定义一个防抖函数debounce。
```html
export default {
methods: {
debounce(func
delay = 200) {
let timer = null;
return function () {
if (timer) {
clearTimeout(timer);
}
timer = setTimeout(() => {
func.apply(this
arguments);
}
delay);
}
}
handleClick: this.debounce(function () {
console.log('按钮点击事件');
})
}
}
```
以上是使用Vue实现防抖的一些方法。防抖技术可以有效地减少重复性的事件触发,提高页面性能。根据实际需求选择合适的实现方式来使用防抖。