vue对象添加属性
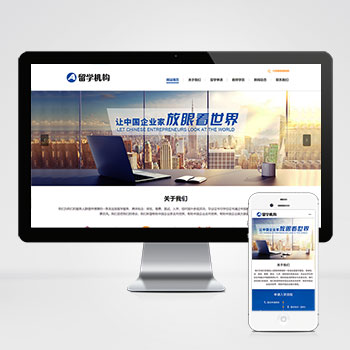
要给vue对象添加属性,我们需要使用Vue.set()方法或者使用$set()方法。
Vue.set()方法的用法如下:
Vue.set(object
key
value)
其中,object是要添加属性的vue对象,key是要添加的属性名,value是要添加的属性值。
举个例子:
```
{{ message }}
export default {
data() {
return {
message: 'Hello
Vue!'
}
}
methods: {
addProperty() {
// 给vue对象添加新的属性
Vue.set(this
'newProperty'
'This is a new property.');
}
}
}
```
上面的例子中,我们在vue对象中添加了一个名为newProperty的新属性,并赋予它一个初始值"This is a new property."。
$set()方法的用法与Vue.set()方法相同,只是$set()方法是在vue对象的实例上调用的。同样的例子可以修改如下:
```
{{ message }}
export default {
data() {
return {
message: 'Hello
Vue!'
}
}
methods: {
addProperty() {
// 给vue对象添加新的属性
this.$set(this
'newProperty'
'This is a new property.');
}
}
}
```
使用$set()方法与使用Vue.set()方法效果是一样的,只不过$set()方法是在vue实例上调用的。
需要注意的是,当我们给vue对象添加新属性时,vue不能检测到这个改动,因此需要使用Vue.set()方法或$set()方法来触发vue的响应式系统。
在实际开发中,我们可能会遇到需要添加多个属性的情况,如果每次添加一个属性都使用Vue.set()方法或$set()方法会显得很麻烦。这时,我们可以使用循环语句来批量添加属性。
举个例子:
```
{{ message }}
export default {
data() {
return {
message: 'Hello
Vue!'
}
}
methods: {
addProperties() {
// 给vue对象添加多个属性
const properties = {
property1: 'This is property 1.'
property2: 'This is property 2.'
property3: 'This is property 3.'
};
for (let key in properties) {
this.$set(this
key
properties[key]);
}
}
}
}
```
上面的例子中,我们使用循环语句给vue对象添加了三个新属性,分别为property1、property2和property3。
总结一下,要给vue对象添加属性,我们可以使用Vue.set()方法或者$set()方法。如果需要批量添加属性,我们可以使用循环语句来实现。虽然这只是一个简单的例子,但在实际开发中,添加对象属性是非常常见的操作,希望这篇文章能帮到你。