vuedata
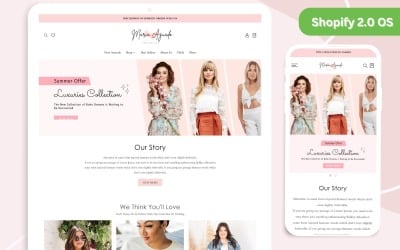
Vue Data
Vue.js is a JavaScript framework that is used for building user interfaces. It focuses on the view layer of an application and provides data-driven views.
In Vue
data is an important concept. It is used to store and manage the state of the application. Vue provides several options for defining and manipulating data.
The simplest way to add data to a Vue component is by using the `data` option. This option accepts a function that returns an object. The properties of this object will be the data properties of the component. For example:
```
data() {
return {
message: 'Hello
Vue!'
count: 0
}
}
```
In this example
the `message` property is a string and the `count` property is a number. These properties can be accessed in the template of the component using the double curly brace syntax `{{ }}`. For example:
```
{{ message }}
Count: {{ count }}
```
When the component is rendered
Vue will substitute the values of the data properties in the template.
In addition to the `data` option
Vue provides the `computed` option for defining computed properties. Computed properties are properties that are derived from the data properties. They can be used to perform calculations or transformations on the data. Computed properties are cached based on their dependencies
which means that they are only recalculated when their dependencies change. For example:
```
computed: {
doubledCount() {
return this.count * 2;
}
}
```
In this example
the `doubledCount` computed property multiplies the value of the `count` data property by 2.
Another option provided by Vue is the `watch` option. The `watch` option can be used to perform side effects when a data property changes. It accepts an object where the keys are the data properties to watch and the values are the functions to be called when the properties change. For example:
```
watch: {
count(newValue
oldValue) {
console.log(`Count changed from ${oldValue} to ${newValue}`);
}
}
```
In this example
the function will be called whenever the `count` property changes. The new value and the old value of the property will be passed as arguments to the function.
In addition to these options
Vue also provides a way to share data between components using the `props` option. The `props` option allows a parent component to pass data to a child component. The child component can then use this data in its template or computed properties. For example:
```
// Parent component
// Child component
props: ['message'
'count']
```
In this example
the `message` and `count` properties of the parent component are passed to the child component using the `props` option.
In conclusion
data is an important aspect of Vue.js. It allows developers to store and manage the state of their applications. Vue provides various options for defining and manipulating data
such as the `data`
`computed`
`watch`
and `props` options. These options make it easy to build reactive and data-driven views in Vue applications.