vue对象转数组
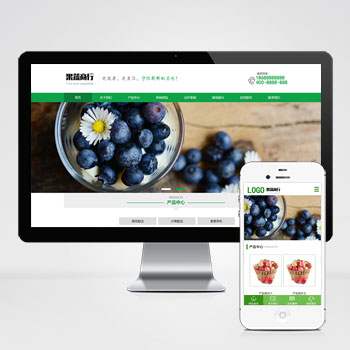
Vue.js 是一个流行的 JavaScript 框架,用于构建用户界面。在 Vue 中,组件的数据可以使用对象的形式进行存储和操作。但在某些情况下,我们可能需要将 Vue 对象转换为数组,以实现不同的需求。在本文中,我将讨论几种常见的方法,以实现 Vue 对象到数组的转换。
1. 使用 Object.values() 方法:
Object.values() 方法返回一个给定对象的所有可枚举属性值的数组。我们可以使用此方法将 Vue 对象的属性值提取出来,并存储到一个新的数组中。以下是一个示例代码:
```javascript
// 创建一个 Vue 对象
const vm = new Vue({
data: {
name: 'John Doe'
age: 25
email: 'johndoe@example.com'
}
})
// 将 Vue 对象转换为数组
const arr = Object.values(vm.$data)
console.log(arr) // 输出:['John Doe'
25
'johndoe@example.com']
```
2. 使用 Object.entries() 方法:
Object.entries() 方法返回一个给定对象的所有可枚举属性的键值对数组。我们可以使用此方法将 Vue 对象的属性和值以键值对的形式提取出来,并存储到一个新的数组中。以下是一个示例代码:
```javascript
// 创建一个 Vue 对象
const vm = new Vue({
data: {
name: 'John Doe'
age: 25
email: 'johndoe@example.com'
}
})
// 将 Vue 对象转换为数组
const arr = Object.entries(vm.$data).map(([key
value]) => ({ key
value }))
console.log(arr)
// 输出:[
// { key: 'name'
value: 'John Doe' }
// { key: 'age'
value: 25 }
// { key: 'email'
value: 'johndoe@example.com' }
// ]
```
3. 使用 lodash 库:
借助于 lodash 库,我们可以更简便地实现 Vue 对象到数组的转换。使用 lodash 的 `_.toArray` 方法,我们可以将 Vue 对象转换为数组。以下是一个示例代码:
```javascript
// 引入 lodash 库
import _ from 'lodash'
// 创建一个 Vue 对象
const vm = new Vue({
data: {
name: 'John Doe'
age: 25
email: 'johndoe@example.com'
}
})
// 将 Vue 对象转换为数组
const arr = _.toArray(vm.$data)
console.log(arr) // 输出:['John Doe'
25
'johndoe@example.com']
```
以上是三种常见的方法,可以实现 Vue 对象到数组的转换。根据实际需求选择合适的方法进行使用。希望这篇文章对您有所帮助!