vue装饰器
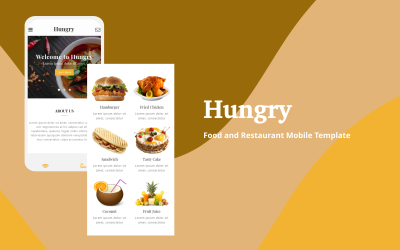
Vue装饰器是一种语法糖,可以在Vue组件中简化代码的书写和逻辑处理,提高开发效率和代码的可维护性。在Vue.js 2.0之前,Vue并未原生支持装饰器,但通过第三方库,如vue-class-component和vue-property-decorator,可以在Vue组件中使用装饰器。
Vue装饰器常用的有三种:@Component、@Prop和@Watch,分别用于标识组件、定义组件的属性和监听属性的变化。
@Component装饰器用于标识一个Vue组件,可以通过它来定义组件的模板、样式、生命周期钩子函数等。使用@Component装饰器可以简化组件的定义,将组件的模板、样式和脚本集中在一起,提高代码的可读性和维护性。例如:
```
import { Component
Vue } from 'vue-property-decorator';
@Component({
template: `
{{ message }}
`
style: `
p {
color: blue;
}
`
})
export default class MyComponent extends Vue {
message = 'Hello World!';
showAlert() {
alert(this.message);
}
}
```
@Prop装饰器用于定义组件的属性,将属性传递给子组件。使用@Prop装饰器可以方便地定义和传递属性,并在子组件中使用。例如:
```
import { Component
Prop
Vue } from 'vue-property-decorator';
@Component({
template: `
{{ message }}
`
})
export default class ChildComponent extends Vue {
@Prop() message!: string;
}
```
@Watch装饰器用于监听属性的变化,在属性发生变化时执行相应的逻辑。使用@Watch装饰器可以方便地监听属性变化,并在变化时执行相应的业务逻辑。例如:
```
import { Component
Prop
Vue
Watch } from 'vue-property-decorator';
@Component({
template: `
{{ message }}
`
})
export default class MyComponent extends Vue {
@Prop() message!: string;
updatedMessage = '';
@Watch('message')
onMessageChanged(newValue: string
oldValue: string) {
console.log(newValue
oldValue);
this.updatedMessage = newValue + ' updated';
}
}
```
除了以上三种装饰器,还可以使用其他装饰器来简化代码的书写,如@Emit、@Inject、@Provide等。在使用这些装饰器时,需要在Vue组件中的装饰器前面加上@前缀,表示使用装饰器语法。同时,需要将组件的脚本文件设置为TypeScript或TypeScript+装饰器相关插件的编译环境,以支持装饰器的语法。
总结一下,Vue装饰器是一种可以在Vue组件中使用的语法糖,通过简化代码的书写和逻辑处理,提高开发效率和代码的可维护性。常用的装饰器包括@Component、@Prop和@Watch,分别用于标识组件、定义组件的属性和监听属性的变化。除了这些装饰器,还可以使用其他装饰器来简化代码的书写。使用装饰器时,需要在Vue组件中的装饰器前面加上@前缀,并确保组件的脚本文件设置为TypeScript或TypeScript+装饰器相关插件的编译环境。