vue图片
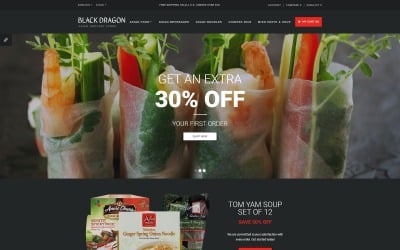
Vue.js is a popular JavaScript framework that is widely used for building user interfaces. One of the key features of Vue.js is its ability to handle images effectively
allowing developers to easily integrate and display images in their Vue applications. In this article
we will explore the various ways Vue.js can be used to work with images and discuss some best practices for optimizing image usage in Vue projects.
One of the basic requirements when working with images in Vue.js is to import the desired image into the project. This can be done using the `require()` function
which allows you to import the image file into your Vue component. By doing so
the image file will be processed and included in the bundle created by the Vue build process. For example
let's say we have an image file called "example.png" that we want to import into a Vue component. We can do this by using the following code:
```
export default {
data() {
return {
exampleImage: require('../assets/example.png')
}
}
}
```
In the above code
we are using the `require()` function to import the "example.png" image file from the "../assets" directory. We then assign the imported image to a data property called `exampleImage`. Finally
we use the `:src` binding to dynamically set the `src` attribute of the `` tag to the value of `exampleImage`.
Vue.js also provides a convenient way to dynamically load images based on conditions or user interactions. For example
you may want to load different images depending on the user's actions or the state of your application. To achieve this
you can bind the `:src` attribute of the `` tag to a computed property or a method that dynamically returns the URL of the desired image. Here's an example:
```
export default {
data() {
return {
image: 'example1.png'
}
}
computed: {
getImage() {
return require(`../assets/${this.image}`);
}
}
methods: {
changeImage() {
this.image = 'example2.png';
}
}
}
```
In the above code
we have a button that triggers the `changeImage` method
which updates the `image` data property to a different image filename. The `getImage` computed property uses string interpolation to dynamically generate the URL of the image based on the `image` data property. Thus
whenever the `image` property changes
the computed property is re-evaluated
and the corresponding image is loaded.
When working with images in Vue.js
it is also important to consider the performance aspects. Large or high-resolution images can significantly impact the loading time of your Vue application. To optimize image usage
it is recommended to use images in the appropriate size and format. Additionally
compressing images can help reduce their file size without compromising their quality. There are several tools available for image compression
such as `imagemin` or `tinyjpg`
which can be integrated into your build process to automatically optimize images.
Another optimization technique is lazy loading
where images are loaded only when they are about to enter the user's viewport. This can help improve the initial loading speed of your application
as images that are not immediately visible to the user are not loaded until needed. There are various Vue plugins available
such as `vue-lazyload`
which provide easy-to-use lazy loading functionality.
Finally
when hosting your Vue.js application
it is recommended to leverage content delivery networks (CDNs) that specialize in serving static assets
such as images. CDNs help distribute your images across multiple servers worldwide
reducing the latency and improving the overall loading speed of your application.
In conclusion
Vue.js provides a rich set of options for working with images in Vue applications. By importing images using the `require()` function
dynamically loading images based on conditions or user interactions
and optimizing image usage for performance
you can effectively manage and display images in your Vue projects.