vue跨域前端怎么解决
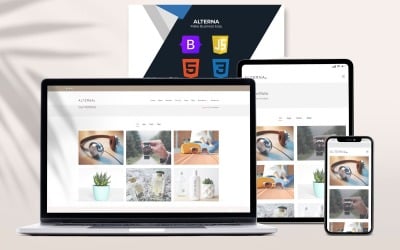
跨域问题是指浏览器的同源策略限制了一个网页从一个源(协议+域名+端口)去请求另一个源的资源。在前端开发中,我们经常会遇到需要解决跨域问题的情况。本文将从以下几个方面详细介绍如何解决Vue跨域问题:
1. 理解同源策略:
同源策略(Same-Origin Policy)是浏览器的一种安全策略,它限制了一个网页从一个源去请求另一个源的资源。同源是指两个URL拥有相同的协议、域名和端口号。同源策略的存在,可以有效防止恶意网站窃取用户信息等安全问题。
2. 解决跨域问题的方法:
在Vue中,我们可以通过代理、JSONP、CORS和反向代理等方式来解决跨域问题。
2.1 代理:
在开发环境中,我们可以通过配置代理服务器(proxy server)来解决跨域问题。在Vue中,可以使用devServer.proxy配置来实现代理。具体步骤如下:
(1)在vue.config.js文件或webpack配置文件中,配置devServer.proxy:
```
module.exports = {
devServer: {
proxy: {
'/api': { // 接口的前缀
target: 'http://localhost:3000'
//需要跨域的服务器地址
pathRewrite: {
'^/api': '' // 重写路径
}
changeOrigin: true // 支持跨域
}
}
}
}
```
(2)在需要跨域的请求中,使用相对路径或带有前缀“/api”的URL:
```
axios.get('/api/users')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
})
```
2.2 JSONP:
JSONP(JSON with Padding)是一种跨域解决方案,它利用了
```
(2)在页面中定义回调函数:
```
function handleResponse(data) {
console.log(data);
}
```
2.3 CORS:
CORS(Cross-Origin Resource Sharing)是一种现代浏览器支持的跨域解决方案。在服务器返回的响应头中添加Access-Control-Allow-Origin字段,设置允许跨域的域名即可。具体步骤如下:
(1)在服务器返回的响应头中添加Access-Control-Allow-Origin字段,设置允许跨域的域名。例如,在Node.js中可以使用cors库:
```
const express = require('express');
const cors = require('cors');
const app = express();
app.use(cors());
app.get('/api/users'
(req
res) => {
res.json({name: 'Alice'
age: 20});
});
app.listen(3000
() => {
console.log('Server is running on port 3000');
});
```
(2)在Vue中发送跨域请求:
```
axios.get('http://localhost:3000/api/users')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
})
```
2.4 反向代理:
反向代理是一种常见的跨域解决方案,它通过在服务器端设置代理服务器来实现跨域请求。具体步骤如下:
(1)在服务器端配置反向代理服务器,转发请求到目标服务器。例如,可以使用Nginx服务器:
```
server {
listen 80;
server_name example.com;
location /api {
proxy_pass http://api.example.com;
proxy_set_header Host $host;
proxy_set_header X-Real-IP $remote_addr;
}
}
```
(2)在Vue中发送请求到代理服务器:
```
axios.get('/api/users')
.then(response => {
console.log(response.data);
})
.catch(error => {
console.log(error);
})
```
3. 注意事项:
跨域解决方案在不同的场景下有不同的适用性。在开发阶段,使用代理或JSONP是比较方便的解决方案。在生产环境中,使用CORS或反向代理是更合适的选择。
总结:
跨域问题在前端开发中经常遇到,为了解决这个问题,我们可以使用代理、JSONP、CORS和反向代理等方法。每种方法都有其适用的场景和注意事项。通过理解同源策略和选择合适的解决方案,我们可以轻松地在Vue中解决跨域问题。